Innovate
Your Software
Faster without Risk
Innovate Your Software Faster without Risk
How to Implement Advanced Feature Flagging Techniques in C# Applications
Introduction
Feature flags, also known as feature toggles, are a powerful tool in software development that allow developers to enable or disable certain features or code paths within an application. Feature flags can be utilized in a variety of ways:
- They can be environment-specific, enabling a feature for testing in a Q&A environment while keeping it disabled in production until it's fully ready.
- They can be user-specific, allowing a feature to be rolled out to a particular user or user group, such as testers.
- They can be time-specific, enabling a feature to be rolled out at a future date or for a specific duration, like displaying a banner.
Some common use cases for feature flags include:
- Rollouts - Gradually ramp % of users who see new features
- Kill Switches - Instantly disable faulty features
- Experimentation - Run A/B tests to decide which variant wins
- Dark Launches - Test new features in production without exposing them
- Configuration - Adjust application settings based on environmental factors or user attributes
In this article, we will dive into the implementation of advanced flagging techniques. These techniques include precise targeting, data-driven releases, context-based activation, and centralized configuration. We will also demonstrate these techniques within the context of a basic web application, utilizing open source feature flags tool FeatBit for the creation and management of feature flags.
Understanding Feature Flagging
Before delving into advanced techniques, let's revisit the fundamentals of feature flagging. At its core, feature flagging involves using conditional statements in code to dynamically control a feature's behavior based on certain conditions, such as user attributes or environmental factors.
Let's consider a simple example using a boolean flag named use-new-welcome-message
:
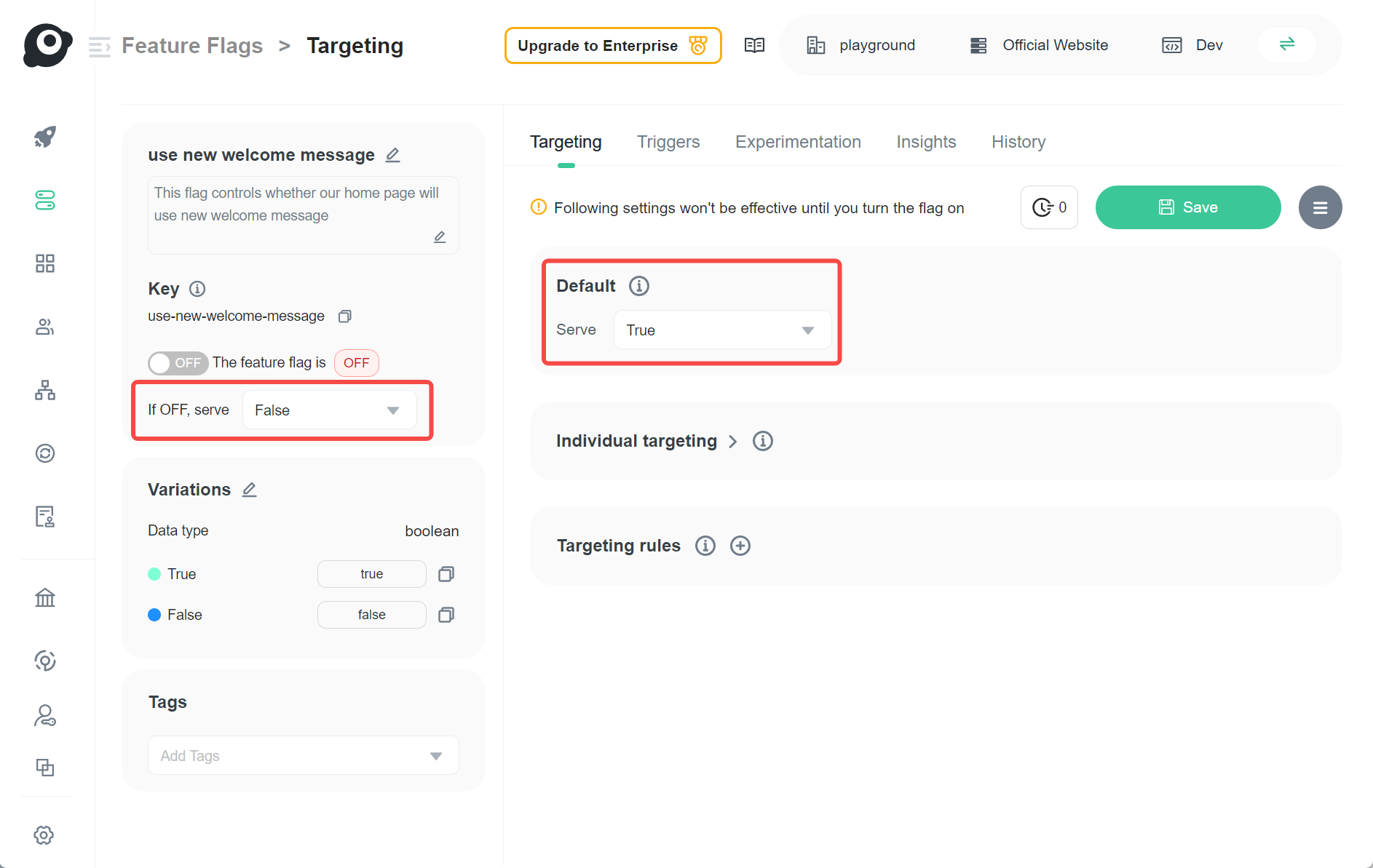
In the above image, the flag use-new-welcome-message
is depicted with a boolean value. When enabled, it
returns true
;
otherwise, it returns false
. This flag can be utilized in code to determine which welcome message to display:
public void OnGet()
{
WelcomeMessage = _fbClient.BoolVariation("use-new-welcome-message", userContext, defaultValue: false)
? "New Welcome!"
: "Welcome!";
}
Another approach is to treat the feature flag as a configuration setting, directly incorporating its value into the
code. Let's examine an example using a string flag named welcome-message
:
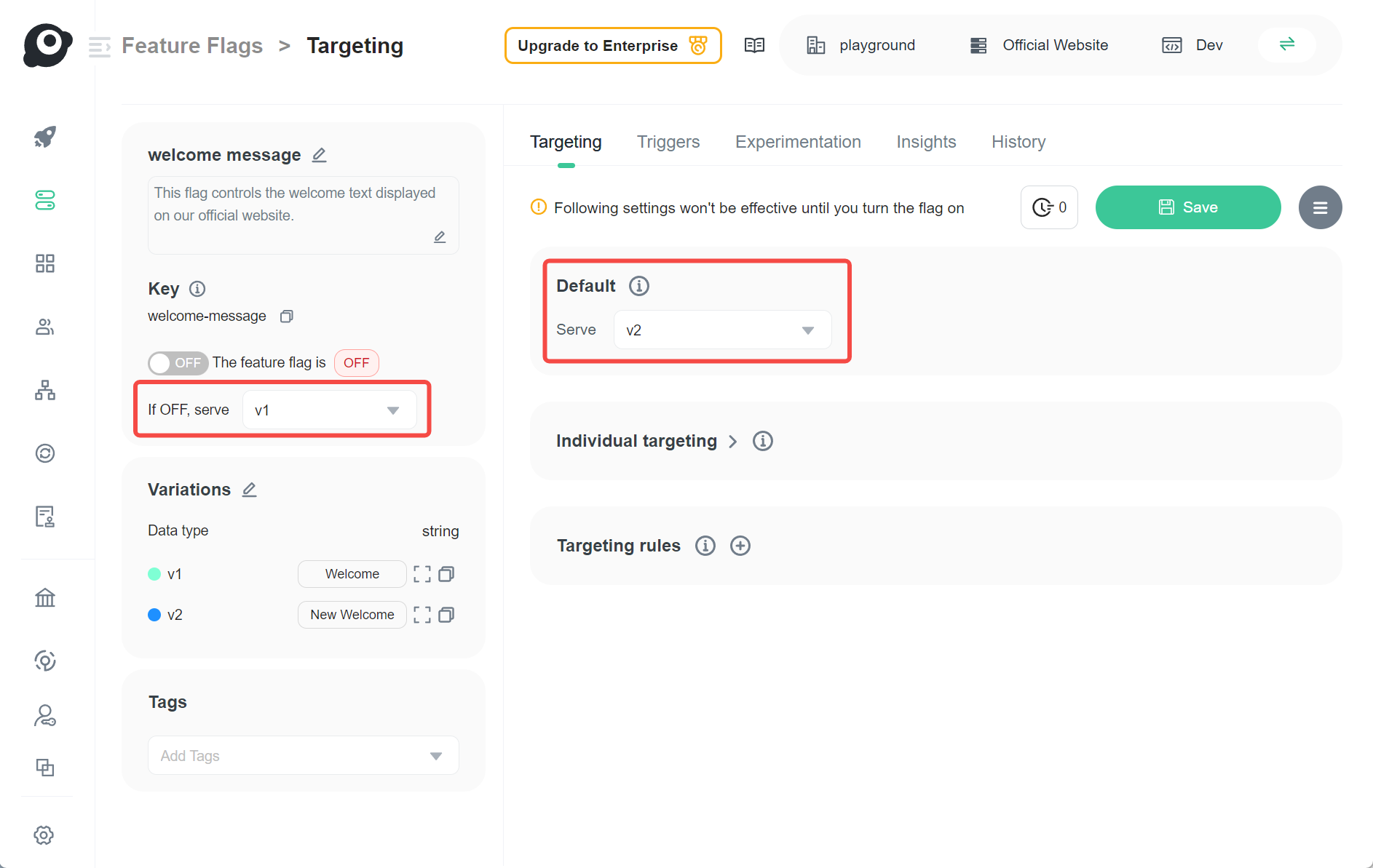
In the image above, the flag welcome-message
is presented with a string value. When enabled, it serves the v2
value,
which is "New Welcome"; otherwise, it serves the v1
value, "Welcome".
And then in your code, you can do this:
public void OnGet()
{
WelcomeMessage = _fbClient.StringVariation("welcome-message", userContext, defaultValue: "Welcome!");
}
In both examples, if the flag is enabled, the new welcome message will be displayed; otherwise, the old one will be shown.
Segmentation and Targeting
Segmentation involves dividing users into distinct groups based on various attributes such as demographics, behavior, or preferences. Feature flags can be leveraged to target specific segments for controlled rollouts or experiments, allowing developers to gather targeted feedback and insights. For example, We can define user segments based on attributes like:
- Email patterns (e.g. @microsoft.com)
- Sign-up dates (e.g. users Pre 2024)
- Subscription plans (e.g. premium customers)
Precise targeting allows you to control your feature based on specific user attributes. This strategy is particularly beneficial for A/B testing scenarios, where a new feature needs to be exposed to a specific user group for testing before a full-scale rollout. With FeatBit, precise targeting can be implemented via:
- Individual Targeting: This allows you to allocate specific flag variations to individual users.
- Targeting Rules: This enables you to establish rules based on user attributes, such as email, name, or role.
Gradual Rollouts and Canary Releases
Gradual rollouts, also known as phased releases, involve gradually increasing the percentage of users exposed to a new feature over time, for example from 10% to 50% and so on. Canary releases follow a similar approach but focus on releasing features to a small subset of users initially, allowing for early testing and monitoring before full deployment. Feature flags play a crucial role in orchestrating gradual rollouts and canary releases, ensuring smooth transitions and minimizing risks. A practical example would be Gradual Database Migration.
Some common use cases for gradual rollouts and canary releases include:
- New Feature Rollouts: Gradually exposing new features to users for testing and feedback collection
- Infrastructure Changes: Rolling out infrastructure updates to a small subset of users for monitoring and validation
- Performance Testing: Exposing new functionality to a small group of users to monitor performance and stability
Context-Based Activation
Contextual activation enables features to be activated based on specific conditions or user behavior, providing personalized experiences and optimizing engagement. For example, a discount code may only be enabled on Mondays or a new layout may be offered to returning users. Feature flags enable developers to implement contextual activation logic efficiently, enhancing user satisfaction and driving conversion rates.
Some use cases for context-based activation include:
- Geo-Gating: Displaying location-specific content or promotions
- Device Targeting: Tailoring the user experience based on the user's device
- User Behavior: Activating features based on user interactions or preferences
- Time-Based Activation: Enabling features at specific times or dates
Configuration Management
Feature flags can be utilized for dynamic configuration management, enabling applications to adjust settings and behaviors dynamically based on environmental factors or user attributes. This approach allows developers to fine-tune the application's behavior without redeploying the codebase, streamlining maintenance and enhancing flexibility.
Some common use cases for configuration management include:
- User-Specific Settings: Tailoring the user experience based on user attributes, such as language, role, or subscription plan.
- Content Management: Dynamically controlling content based on user attributes or environmental factors.
- Security Policies: Adjusting security policies based on environmental factors or user attributes.
Demonstration
In this demonstration, we'll illustrate how to create and use a string flag to control the welcome text shown on the index page of a .NET web application.
Setting the stage
Firstly, ensure you have access to the FeatBit service. You can either try its online version at TryItOnline or set it up locally by following the instructions provided in the Get Started guide.
Next, create a new .NET web application using the following commands:
dotnet new webapp -au Individual -o RazorDemo
cd RazorDemo
dotnet add package FeatBit.ServerSdk
In the Program.cs file, add the following code to configure the FeatBit service:
using FeatBit.Sdk.Server.DependencyInjection;
// add FeatBit service
builder.Services.AddFeatBit(options =>
{
options.EnvSecret = "<use-your-own-server-secret>";
options.StreamingUri = new Uri("<use-your-own-streaming-uri>");
options.EventUri = new Uri("<use-your-own-event-uri>");
options.LoggerFactory = LoggerFactory.Create(x => x.AddConsole().SetMinimumLevel(LogLevel.Information));
options.StartWaitTime = TimeSpan.FromSeconds(3);
});
Replace the placeholders with your actual FeatBit environment secret and URIs. Refer to the FAQ for obtaining the actual values.
Once configured, start the application, and if you see FbClient successfully started
in your console logs, we're ready
to go next.
Creating a feature flag
Navigate to the FeatBit dashboard and create a new flag named welcome-message, as shown in the following image:
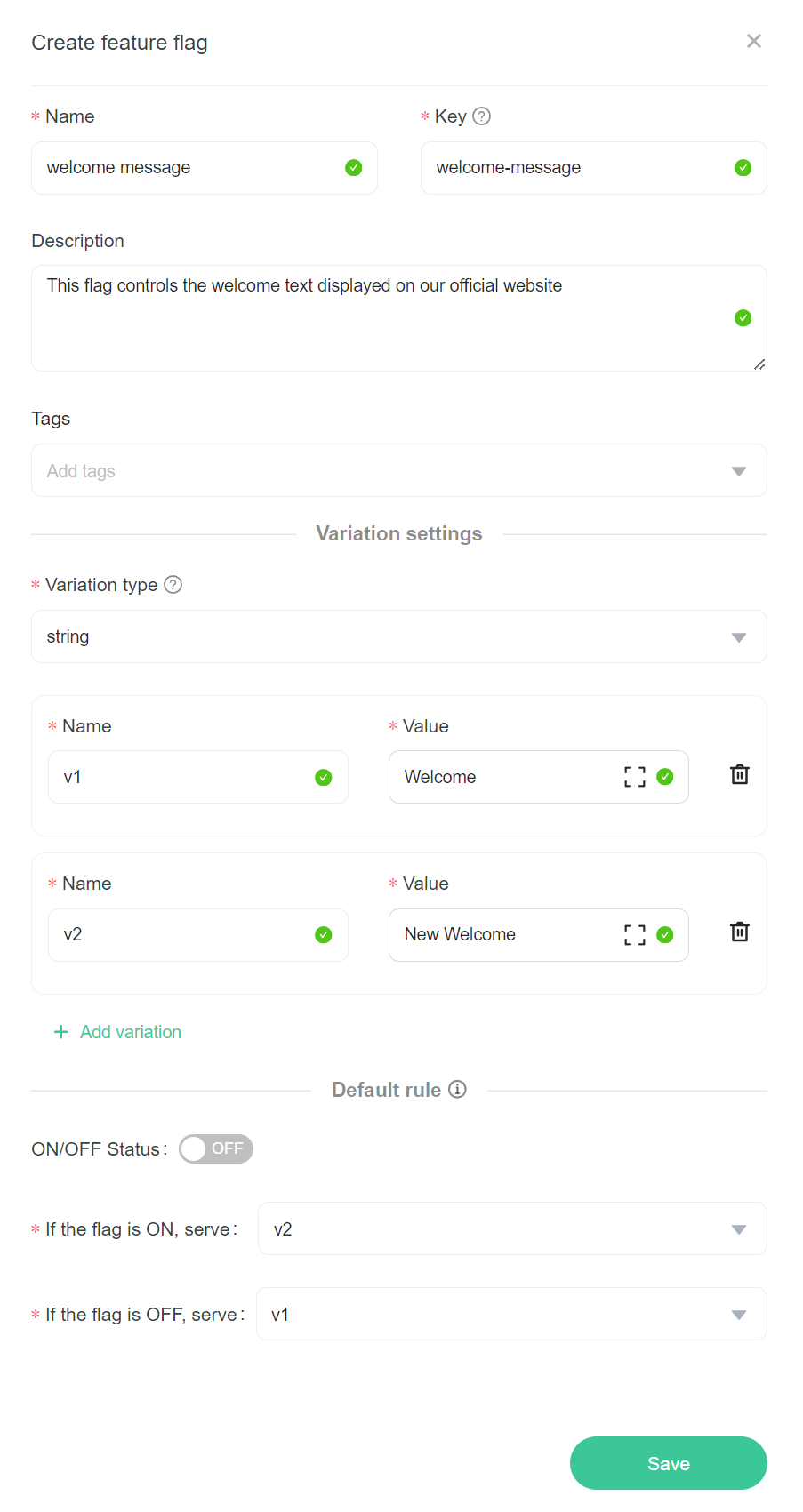
Using the feature flag
Open the Index.cshtml.cs
file and replace its content with the following code:
using FeatBit.Sdk.Server;
using Microsoft.AspNetCore.Mvc.RazorPages;
using RazorDemo.Data;
namespace RazorDemo.Pages;
public class IndexModel : PageModel
{
public string WelcomeMessage { get; set; }
private readonly IFbClient _fbClient;
public IndexModel(IFbClient fbClient)
{
_fbClient = fbClient;
}
public void OnGet()
{
WelcomeMessage = _fbClient.StringVariation("welcome-message", User.AsFbUser(), defaultValue: "Welcome!");
}
}
Then, utilize the WelcomeMessage
property in the Index.cshtml
file:
<h1 class="display-4">@Model.WelcomeMessage</h1>
Now, start the application and navigate to the index page. As the flag is currently in the OFF status, you should see the default welcome message:
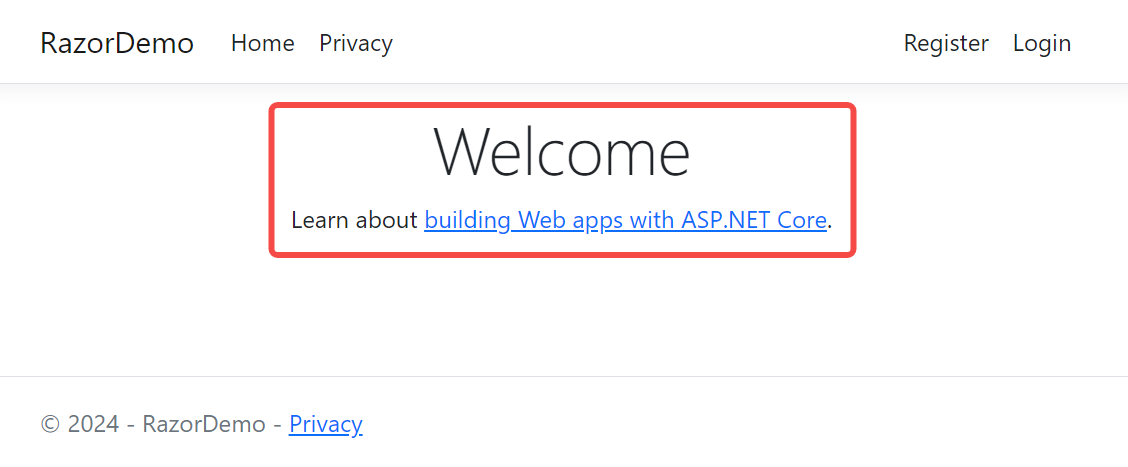
Back to the FeatBit dashboard and turn on the flag, then refresh the index page. You should now see the new welcome message displayed:
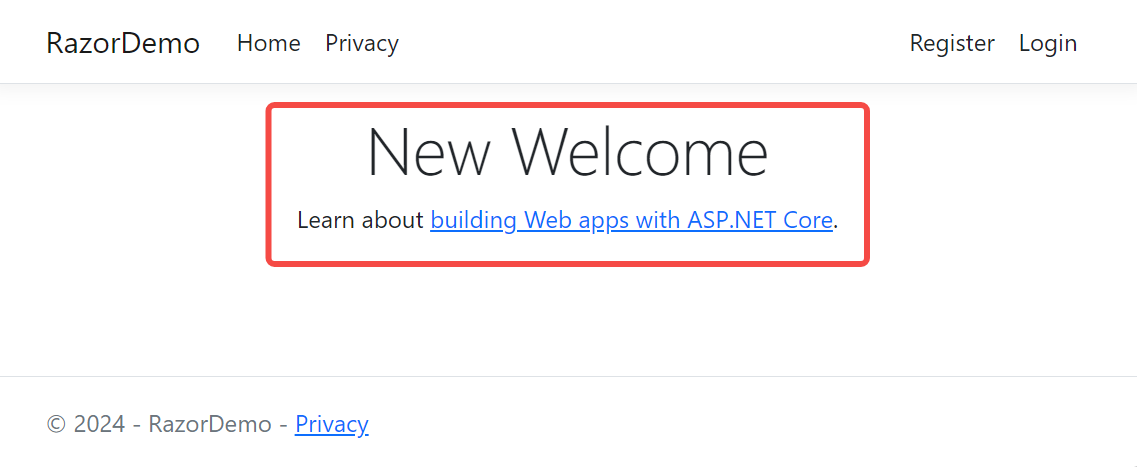
Conclusion
In this guide, we've explored advanced feature flagging techniques in C# applications, unlocking the full potential of feature flags.
- Segmentation and targeting allow precise feature rollouts to specific user groups, enabling effective A/B testing and targeted feedback collection.
- Gradual rollouts and canary releases facilitate smooth deployments by gradually exposing new functionality, minimizing risks.
- Context-based activation offers personalized experiences based on user behavior, optimizing engagement.
- Dynamic configuration management enables on-the-fly adjustments to application settings, enhancing flexibility.
Our demonstration showcased practical application within a .NET web app, using FeatBit for feature flag management. By following these steps, you can integrate advanced feature flagging seamlessly.
To learn more about FeatBit and its feature management capabilities, visit the FeatBit website.